Introduction to C++
C…plus plus a little something something
- Course Length: 3 weeks
- Course Type: Short Course
- Category:
- Life Skills
- Technology and Computer Science
- High School
Schools and Districts: We offer customized programs that won't break the bank. Get a quote.

C is nice, but you know what's better? C++.
No, we aren't talking about a new way of saying you got a B-. We're talking about the incrementation of C into a whole new, fancier language. You'll need some C under your belt before you're ready to start on its slightly younger, much more fashion-forward cousin. Along with giving you tips about what colors to match and which pair of Air Jordans go with your debugging-at-3AM outfit, C++ adds much more built-in functionality through headers and classes.
C++ might be built on C, but it hides so much that you might not even realize it. With
- pointers,
- templates,
- headers and classes,
- arrays and vectors,
you'll be coding in C++ in no time. Now if only understanding why you need a pair of Air Jordans to match your debugging-at-3AM outfit was this easy…
Unit Breakdown
1 Introduction to C++ - Introduction to C++
From memory allocation to templates, we'll cover all the main functionality of C++. The only thing we won't cover is why they couldn't just name it D…
Recommended prerequisites:
Sample Lesson - Introduction
Lesson 1.06: Déjà vu All Over Again
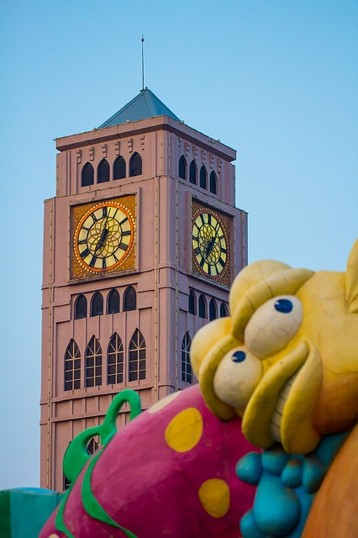
(Source)
We're going to cut to the chase: Your time is precious. (That's why we cut to the chase. You're welcome.)
In the time it takes you to retype necessary code, or even cut and paste it, you could be doing so many other, more worthwhile things, like
- visiting Shijingshan Amusement Park, China's fake Disneyland.
- putting together a jigsaw puzzle.
- putting together a jigsaw puzzle of Shijingshan Amusement Park, China's fake Disneyland.
That's where loops come in.
If you know how to write for, while, and do-while loops, you can make your computer crank out tedious and repetitive tasks for you. With just a few lines of code and the correct structure, your program will run all day and all night, until you tell it to stop, freeing you up to spend as much time as you want reorganizing your sock drawer by odor and planning your trip to Shijingshan Amusement Park, China's fake Disneyland.
Sample Lesson - Reading
Reading 1.1.06a: Making Your Life Easier, One Loop at a Time
You know how you love typing out code and then having to re-type it over and over and over again each time you want to reuse it?
Yeah, us neither.
Fortunately, we have an alternative to typing our fingers raw: loops.
Loops come in three flavors:
- for
- while
- do-while
These three varieties are called iteration statements because they repeat a certain number of times (or for a certain number of iterations) until the conditions are fulfilled.
For the Love of Loops
The odds are pretty slim that you're ever going to need to count to a million. There will be times, however, when you need to repeat a certain action lots and lots and lots of times. When that happens, for loops have your back.
The syntax of a for loop looks like this:
for (initialization; condition; increase/decrease) { statement(s); }
This loop, like the other ones that we we'll meet soon, will continue to repeat statement(s);
block while the conditions hold true. What's cool about for loops is that they allow the programmer to be more specific about exactly how long the loop will run by using counter variables and initializing them at the beginning of the loop itself.
All three sections inside a for loop's parentheses are optional; they aren't necessary as long as you have the correct amount of semicolons. Let's take a closer look at each section.
The initialization
is where you make the initial variable (hence the clever name). This variable is going to be the same from semicolon to semicolon inside the parentheses. In early programming, you'd create a variable like i=0
inside the parents to start your counter off from zero. Like we just said, though, this statement isn't required as long as there's a semicolon present like in our example above…and you've already declared i
.
Next, the condition
phase will be something like i < 10
. As long as i
(your initialization variable) is less than 10, the statement block will be executed. In other words, if the condition value is true, then it'll execute the statements in the loop. If it's false, it'll exit the loop completely.
Finally, the increase/decrease
portion is all about how you want to increment or decrement your initial variable. Any of the following could be thrown into that piece of the loop.
- Multiply it by two (
2 * i
) - Increment it by one (
i++
) - Multiply it by five, subtract two, and then divide by 3
(((i*5)-2)/3)
Probably not that last one—that would be a little bonkers—but you get the point. This section totally depends on your program and its purpose.
Here's an example of a for loop using all the concepts we just went over:
int main(){ //count to 10 for (int i=0; i < 10; i++){ cout << i << " "; } }
Starting at the very beginning, we have the for loop. We initialized the variable to be the variable i
, which is an integer equal to zero. Then we made a condition: as long as our variable is less than ten, we're going to keep outputting the number to the screen. Then we have to increment our variable so that it eventually reaches ten.
There you have it; your first for loop.
Sample Lesson - Reading
Reading 1.1.06b: Come, Sit, Loop for a While
While loops are interesting because, unlike for loops, they don't run on iterations. Instead, a while loop runs based on a true or false statement. As long as the statement's true, the loop will continue, but once it becomes false, it'll terminate the loop.
A while loop looks like this:
while(condition){ statement(s); }
Nice and simple. As long as the condition in parentheses is true, it'll continue to execute the statement over and over and over—until the condition's no longer true.
Say you have the condition set to be that while x == 1
, you want the computer to output the letter a
to the screen, but you never increment or decrement the x-value, so x
will always be 1. Then what'll happen?
Yup. Your loop will run until your computer kicks the bucket. That's why you need to make sure you change your values when using the while loop so your computer isn't sentenced to running that loop for all of eternity. Don't let your while loop turn into—gulp—an infinite loop.
Meet the Do-While Loop
Last but not least, we have the do-while loop. Hopefully, you noticed that this loop has a really similar name to the while loop. Seriously: they're only two letters (and a hyphen) off.
Do-while loops and while loops are extremely similar, but there's a crucial difference. See if you can spot it. Here's what the syntax of a do-while loop looks like:
do { statement(s); } while (condition);
Here's a quick example of a do-while loop:
int x = 0; do { cout << x << " "; x++; }while ( x < 10);
Did you catch the difference? In a do-while loop, the condition isn't evaluated until after the statement is executed. No matter what the condition is, the statement will execute at least once, whereas in a while loop it'll only execute the statement if the condition's met. Aside from that, do-while loops and while loops work exactly the same.
And that's a wrap on this reading loop.
Sample Lesson - Activity
Activity 1.06: The Never-Ending Loop
If a loop ends and no one's around to see it, does it make a print statement? Don't worry; we won't get too philosophical about C++, at least not yet. Instead, let's make some loops and see what happens.
Step One
Open a new project in your IDE.
Step Two
Use your fancy new knowledge of loops to design a never-endingloopthat continuously outputs a letter, number, phrase, or sentence to the screen. When you run the program, it should continue running until you force it to stop by closing the window or stopping to debug. Copy your code into a .txt file and hang on to it; you'll upload later in this activity.
For example, Shmoop might unleash this never-ending loop. Because we're so mature.
#include <iostream> using namespace std; int main() { while (1 < 2){ //Create a mature, sophisticated never-ending loop cout << "Goobers!"; } return 0; }
Step Three
Now that you've become supreme ruler of the never-ending loop—it's a lot like being the ruler of The Never-Ending Story, minus the dated special effects—we'd like you to answer the following two questions for us in one to two sentences each. Go ahead and add these to your .txt file with your code that you created in Step Two and hang on to it. You're going to turn it in after the next step.
- What's the difference between a while loop and a do-while loop?
- If you wanted to create a program that output the numbers 1 – 10 to the screen, which type of loop would you choose and why?
Then move on, Atreyu; there's more to be done on your quest.
Step Four
Rewrite the following code so that the output is "I understand loops".
#include <iostream> using namespace std; int main () { int x = 0; while(x!=0) { cout << "I understand loops" << endl; x++; } return 0; }
Copy your revised code and add it to your .txt file below your never-ending loop and your answers to the two mind-bending questions from Step Three, then upload that bad boy below.
Representing Information Rubric - 25 Points
- Course Length: 3 weeks
- Course Type: Short Course
- Category:
- Life Skills
- Technology and Computer Science
- High School
Schools and Districts: We offer customized programs that won't break the bank. Get a quote.
