Programming: How Does it Work?
Programming: How Does it Work?
How does it work? We can say it in one sentence: it works because we've thought out the algorithm and we know the syntax of the language. Without both of them, we're going to have serious problems. Before you start writing a line of code, you need to establish the problem and how to solve it.
The computer does that, though, doesn't it?
Not even a little. The computer's only as strong as its weakest programmer. It doesn't think; it just computes based on zeros and ones. You need to know how to work out the kinks.
Say you have the problem of organizing the school talent show. We can establish some general guidelines, like a talent can be
Sure, that kid in the back of the class might have a talent for drawing long and winding mazes with his graph paper, but it isn't exactly something you can easily show at a talent show. Without boring the audience, at least.
The main problems are that
- no two acts could come from the same movie.
- you need a good mix of different types of talents to keep people from getting bored.
So let's write an algorithm using a data structure and performance objects.
Here's a simple algorithm:
- Take a performance object.
- If the performance set list doesn't exist yet:
- Make this object the first act in the list.
- Otherwise:
- Check the first and second item in the list. If neither have the same type or source:
- place it in-between them.
- Else: continue through the list until two consecutive items don't have the same type or source.
- If you reach the end of the list without placing the item:
- place it at the end.
- Check the first and second item in the list. If neither have the same type or source:
This isn't perfect, but it'll get us started. Now let's look at the performance object, which has four states.
- Name
- Talent
- Talent type
- Comes from (optional)
We can take three example objects.
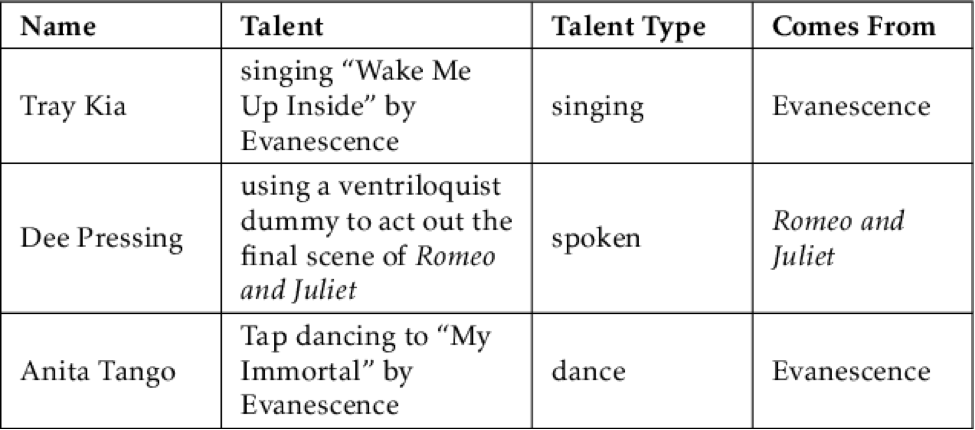
Now that we have our objects, we can put them in a data structure, say a list. We need to head through the list one at a time—constantly adding new nodes—so a linked list is probably our best option.
If we start out with the first object, we can just place it into the linked list:
Tray Kia singing "Wake Me Up Inside" |
Null link |
Then we look at the second option and notice that there aren't any conflicts, so we put it next.
Tray Kia singing "Wake Me Up Inside" | Dee Pressing reenacting Romeo and Juliet |
Dee Pressing | Null link |
Now comes the last one: Anita Tango tap dancing to another Evanescence song. But the first act is also performing Evanescence. If we look at the algorithm, that’s no problem. Now all we need to do is add it to the end of the list.
Tray Kia singing "Wake Me Up Inside" | Dee Pressing reenacting Romeo and Juliet | Anita Tango tap dancing to "My Immortal." |
Dee Pressing | Anita Tango | Null link |
And we have our list of performers. Pretty neat, right?
To sum, in order to get all our programming ducks in a line, we need to figure out
- an algorithm.
- how to separate content into objects and functions.
- which data structures would make the most sense.
Once you have that, all you need to know is the syntax of your language.